
Blackjack Game
I have recently been working on a blackjack game within the program visual studio. It was my first full solo programming project within C++, and I’m reasonably happy with how it turned out in the end. As this was my first C++ project, I have limited knowledge in coding, so I had to work out how I would go about making Blackjack within visual studio. The first thing I did was play a few rounds of the game Blackjack itself, as it had been a while since I had last played the game. As I was playing it, I familiarised myself with the rules and flow of the game, and worked out how I could implement it into C++. I thought out in my head how exactly I could turn it into a game and wrote a few short notes on how I could split it up. I decided that the program would have a menu option, where the user could decide if they wanted to play the game or exit the program.
There would also be a help option if the user needed it. The code would be split into two further sections. These would be setting up the game, and then actually running the game itself. In the setting up of the game, the program would initialise the deck, the player and the dealer. Then, in the second section, the game would actually run, where the user would input their decisions and play the game itself. I knew how I would go about doing the menu system, as this was relatively easy. I could just use a couple of functions and switches in order to allow the user to navigate the menus. What I was most unsure of what I could do, was how I would go about setting up for the game, as I already have a rough idea of what I would do for the game itself. Fortunately, we learned about arrays in lectures, and that this is the best way to set up the deck. Before I started the assignment, I did some further research on arrays by looking over lectures again, and looking at examples of how they were used in other programs online.
Whilst my code went through many variations, I believe the completed version to be the most user friendly, allowing the easiest access and navigation for the user. It also one of the more readable versions, as I spent a large amount of time refining the code to make the sections clear, and made it easy to spot what does what within the program. The first thing the user will be presented with is the main menu. In this, the user is welcomed to the program, and given three very clear options. They can either play the game (this is self-explanatory), chose to receive help, or exit the program entirely. This section of code has stayed regularly similar throughout the development of my code, as it was just as successful in the first tests and is it was in the final checks. Upon selecting for the help option, the console is cleared, and information about Blackjack and how the user plays the game is displayed. It offers advice on how the user can navigate the menus and options, and how they can actually play the game within the program. After a few seconds, the user is able to return to the menu where they can select another option (or ask for help again, if they decide to do this). Aside from playing the game, the user can also select to exit the game from the main menu. Upon selecting this option, the user is thanked for using the program, and then after a few seconds, the program completely closes. The last option the user can select from the main menu is the option to play the game. Upon doing this, multiple things run in the background of the code, which the user cannot see. However, I will focus on what the user can see and do within the program. The player is alerted by the console that they have received two cards from the deck. These cards are random, and change every time the player starts a new game. They are told their first card, and then told their second card immediately afterwards. After receiving knowledge of their first two cards, the user is informed of their total current score, which is the sum of their two cards added up. With this newly received information, the user is then asked if they would like to “Hit or stand” (if they would like to receive another card from the deck, or if they would like to receive no cards and see if their current score is higher than the dealers, but below 21). If they select option one (hit), they will receive another card. The user is told what this card is, and the total sum of all of their cards together. The user is then prompted and asked if they wish to hit or stand again. The user can continue to hit, until they have either got a full hand (six cards), or if they exceed a score of the limit of 21. If they exceed the limit of the 21 by repeatedly hitting, the user will be told that they have lost, and what the dealers score is. They will then be asked if they wish to play again, or exit the game. Exiting the game will close the program, and playing again will bring them to the menu. However, if they decide to stand at any point before having a score of 21 or running out of cards, they will then be informed of what the dealer’s cards are. The program will also then tell them what the total score is of the dealer is, followed by if they have won or lost. The user will then be presented with the option of if they would like to close the game or play again, which I previously explained. This section of code has undergone many iterations, as it is where the user would spend the majority of their time within the program. One of the changes I did to improve the users experience was to change how the options were displayed. In an early version, the user was told that they could simply chose between Hit and Stand with one and two, but I then added a couple more lines which would output “1) Hit”, and “2) Stand”, which looks far nicer and fits with the menu better too.
There is also a large amount of work being done by the program in the background; this has undergone many changes to make it more efficient, to deal with the addition and loss of features, and to comply with the BU coding standard. In the background of the code multiple things are worked out and completed. This includes the starting of the game, the deck, the dealer, and workings out of the player’s cards. The first thing that you can notice within the ‘game’ function is the setting up of the deck via an array. This array was actually originally created by Andrew Watson, however, I had to modify it so it would fit in with the BU coding standard. The main change was within the switch of the array. After the array is set up, the user’s first card is displayed. This is done by using a ‘rand’ command, which randomly picks a number, and is set to pick one between 1 and 52. The number it randomly generates is then used to pull that card from the array. It then uses this information to display what card it is, and the value of it. Next, another card is pulled from the array by using the ‘rand’ command, which is also displayed to the user. The total of these cards is added up, and then displayed to the user. After the user is asked if they want to hit or stand the game checks to see if they are above 21 which would instantly tell them that they have failed, or if they are dead on 21, which grants them an instant victory. This is checked every time the player ‘hits’. The victory and failure messages are in a separate function, to try and help to keep things tidier. If the user choses to hit, the program fetches another card from the array using the rand command again and instantly tells them the card and their total. This cycle continues until the user either hits 21 (a victory), goes over 21 (a loss), runs out of cards, or stands. The dealer’s cards are fetched and displayed in the same way as the player receives their cards, as are the totals. The totals are compared, and whoever has the greater score wins. After the player has won or lost, they are given the choice between exiting the program and playing again. Selecting exit will change the value of ‘exit’, making the program to close. Selecting the play again option will allow the user to return to the main menu where they can play again, select help, or exit from there. Exiting from the main menu actually looks slightly better in terms of the user, as it shows a message to the user for a few seconds, whilst selecting exit after a loss just closes the program nearly instantly. This would be one of the changes I would have made if I were to remake the blackjack program again. The entire program went through many variations, with each making numerous changes. One challenge I found whilst coding, was the coding standard. I had to change a large amount of my work in order to try and fit with the coding standard, and I spent a good hour or so ensuring that it complied with the coding standard. Unfortunately, with limited time to go, I had to redesign my entire code due to an error with my PC. However, I used this as an opportunity to try and make my blackjack as efficient and streamlined as possible, however, it meant I lost out on a couple of features. For example, in older versions, the game function would loop, due to a while loop, which would only cancel when the user chose to exit (similar to that of the one on my menu). This meant that exiting the game actually would bring the user back to the menu first, instead of actually closing the game straight away. Whilst I am disappointed that I ended up cutting features out, it did mean that I ended up with a very streamlined game that is the original blackjack. If I made the game again, I would re-add these features and possibly more. I would also try and seek out others that could review the code, and give me advice on what changes I could make.
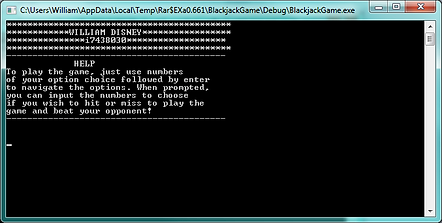

